To maximize the efficiency of solar panels, pyranometers are used to measure solar irradiance under a variety of conditions. Solar irradiance is a measure of power per square meter, and depends on factors like the time of day and the direction of a solar panel. As with any energy source, efficiency is an important consideration since it allows us to do more with less. This project uses a solar cell, a simple amplifier circuit, a voltmeter, and the STEMBoT to measure the power output of the solar cell, which is then used to calculate irradiance.
To begin, the maximum voltage of the solar cell with a load (resistor) attached should be tested and recorded. This will be helpful in designing the amplifier circuit, since the output of the circuit cannot exceed the input of the STEMBoT’s analog-to-digital converter (ADC). To measure the maximum voltage, I selected a resistor of 100 Ohms and placed it in series with the solar cell. Then, while flashing the brightest flashlight I could find directly over the cell, I took voltage readings.
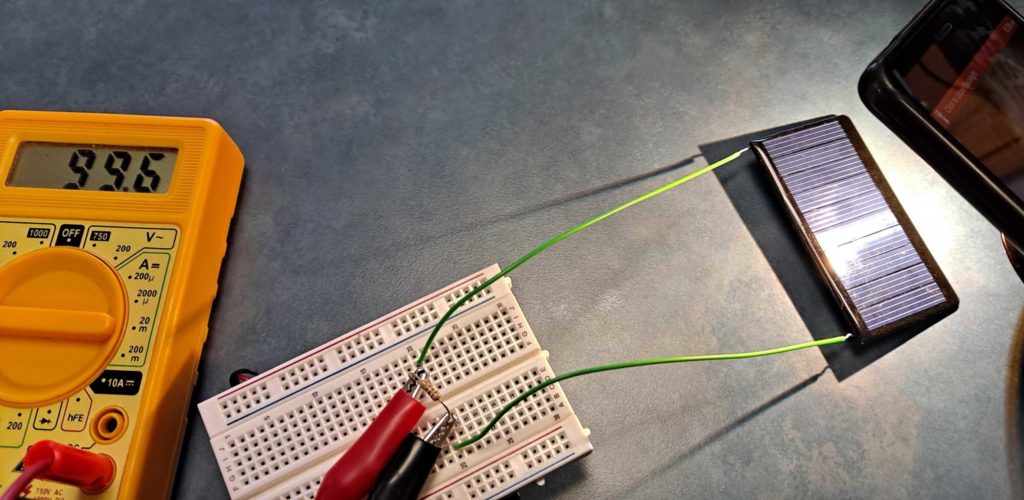
With a maximum voltage of (roughly) 100mV and a maximum input to the ADC of 3.3V, I know through simple division that the amplifier’s gain should be no more than 33. [The gain of a DC voltage is just the output divided by the input]
The next step is to design the amplifier circuit with this gain in mind. For this, an operational amplifier (op-amp) can be used with a basic non-inverting amplifier circuit. I chose an MCP-601 for the op-amp since it can be used with the 3.3V supply the STEMBoT offers. The non-inverting amplifier configuration requires two resistors to define the gain according to the equation in the following schematic.
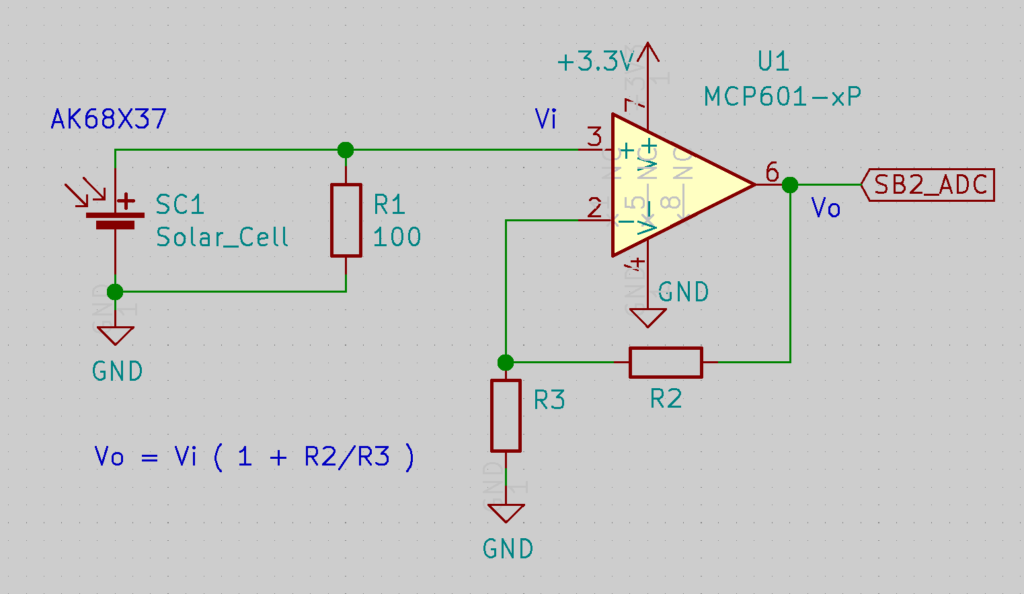
I used a 2.2kOhm resistor for R2 and an 82 Ohm resistor for R3 for a gain of about 27.8. While not ideal, the most important thing is that the gain doesn’t exceed 33 for this setup. It’s okay if the maximum output of the op-amp is below 3.3V, but it cannot be above.
If you’re using a different op-amp, you should consult the respective datasheet to determine how the pins are configured, but in the end this is what my pyranometer looks like.
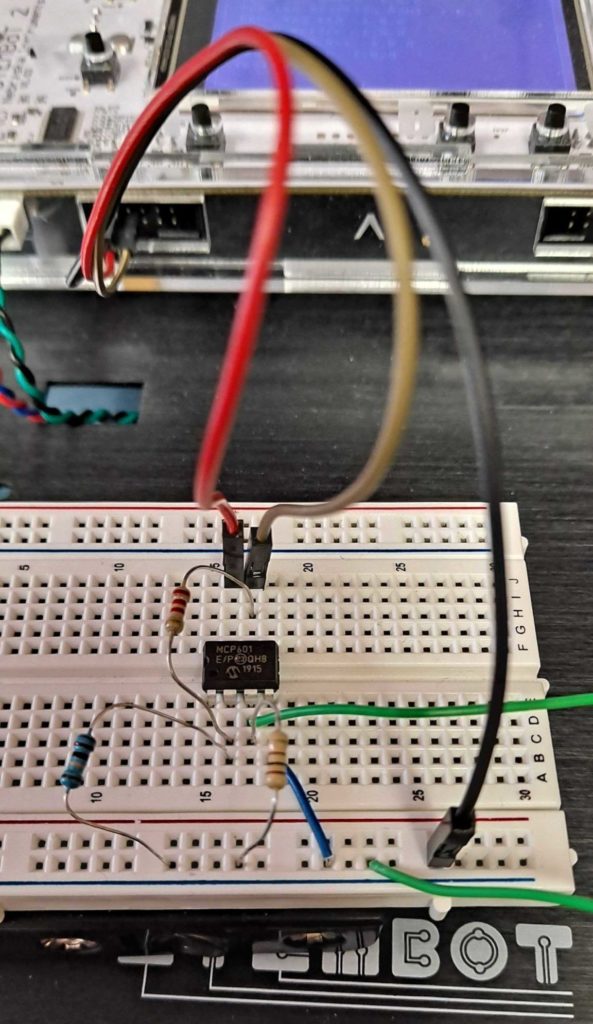
The reading on the ADC will give a number between 0 and 65536 or 2^16. This reading represents a fraction of the maximum voltage (3.3V). For instance, a reading of 0 will indicate 0V on the ADC, and a reading of 65536 would indicate 3.3V. A reading of 32768 is halfway between 0 and 65536, and would represent 1.65V, or halfway between 0V and 3.3V. To find the voltage on the pin based on other readings, take the reading divided by the maximum on the ADC, and multiply it by 3.3V.
ADC_reading/ADC_max = V_reading/V_max
so, V_max * ADC_reading/ADC_max = V_reading
That reading will have to be divided by the gain to get the voltage out of the solar cell.
To find the power based on the voltages, well have to use Ohms Law, V=IR, where V is the voltage, I is the current, and R is the resistance. First find the current going though the load resistor by dividing V by R. Then to find power, multiply V and I. To find irradiance, divide this power by the surface area of the solar cell. In this example, I’m using a 68mm x 37mm cell. The total surface area is then 0.002516m^2. Calculating these values using Python should look something like this:
>>>import machine
>>>import utime
>>>ADC=machine.ADC(“C4”)
>>>ADC_max=2**16
>>>V_max=3.3
>>>gain=27.8
>>>R=100
>>>surface_area=0.002516
>>>while(True):
. . . utime.sleep_ms(500)
. . . ADC_reading = adc.read_u16()
. . . V = V_max * ADC_reading/ADC_max / gain
. . . I = V / R
. . . P = I * V
. . . irradiance = P / surface_area
. . . print(str(irradiance))
This code will print out the solar irradiance every half second. Chances are unless you’re programming in a very bright room, the value returned will be very small. If you shine a light directly over the cell, the irradiance will increase, and if you move your hand over the cell it should fall to zero.
While you won’t be able to power your STEMBoT with solar power directly (yet!) this is still a useful tool for figuring out where you should point any solar panels for making the most of them.